In a typical webpage, images are not embedded in the HTML code, but stored as separate files on the server and requested by individual HTTP GET commands. For different reasons it would be useful to include images in the main web document :
- to speed up the rendering of the webpage in the browser : 80% of the end-user response time is spent on the front-end by downloading all the components in the page: images, stylesheets, scripts, Flash, etc
- to minimize HTTP requests and to reduce trafic costs (e.g. when using an Amazon webservices pricing model)
- to display images created by code (e.g. sparklines)
- to display images retrieved from a database or from a REST service
In august 1998, L. Masinter from Xerox Corporation published a Request for Comment (RFC 2397) for a Uniform Resource Locator (data:URI) scheme that provides a way to include data in line in web pages as if they were external resources. Although the IETF never formally adopted it as a standard, the HTML 4.01 specification refers to the data URI scheme and data URIs have been implemented in most browsers, but not in Internet Explorer before version 8, which is the major problem of this solution.
An example of a red dot picture embedded in HTML is given hereafter :
<img src="data:image/png;base64, iVBORw0KGgoAAAANSUhEUgAAAAoAAAAKCAYAAACNMs+9AAAABGdBTUEAALGP C/xhBQAAAAlwSFlzAAALEwAACxMBAJqcGAAAAAd0SU1FB9YGARc5KB0XV+IA AAAddEVYdENvbW1lbnQAQ3JlYXRlZCB3aXRoIFRoZSBHSU1Q72QlbgAAAF1J REFUGNO9zL0NglAAxPEfdLTs4BZM4DIO4C7OwQg2JoQ9LE1exdlYvBBeZ7jq ch9//q1uH4TLzw4d6+ErXMMcXuHWxId3KOETnnXXV6MJpcq2MLaI97CER3N0 vr4MkhoXe0rZigAAAABJRU5ErkJggg==" alt="Red dot" />
Data URIs may contain whitespace for readability.
Another way to embed images in HTML code is the new element <canvas> which is part of HTML5 and allows for dynamic scriptable rendering of bitmap images. A tutorial about the canvas tag is available at the Mozilla developer center. As for the data:uri, the canvas tag is not supported by Internet Explorer. ExplorerCanvas developed by Erik Arvidsson brings the same functionality to Internet Explorer. To use this solution, web developers only need to include a single script tag in their existing web pages.
<head><!--[if IE]><script src="excanvas.js"></script><![endif]--></head>
To get the code working, the following additional javascript is needed :
var el = document.createElement('canvas'); G_vmlCanvasManager.initElement(el);var ctx = el.getContext('2d');
The classic solution to display binary images embedded in HTML code is the following :
<html> <body> <img src="myimage.php"> </body> </html>
The corresponding code for myimage.php is :
<?php header('Content-type: image/jpeg'); // Create image $image = imagecreatetruecolor($width,$height); // pick color for the background $bgcolor = imagecolorallocate($image, 100, 100, 100); // fill in the background with the background color imagefilledrectangle($image, 0, 0, $width, $height, $bgcolor); // output image to the browser imagejpeg($image); // delete the image resource imagedestroy($image);
Be sure that there is no leading whitespace or blank line before the <?php tag, otherwise the displayed result will be of the form
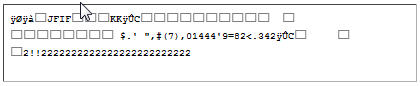
Garbage
One of the first cross-browser methods for incorporating images into self-contained HTML documents was published by Benn P. Herrera in march 2005. Two years ago there was not yet a better method avilable. Today the <canvas> tag and the ExploreCanvas JavaScript seems to be the most promising solution to embed binary images in HTML code.